Buttons¶
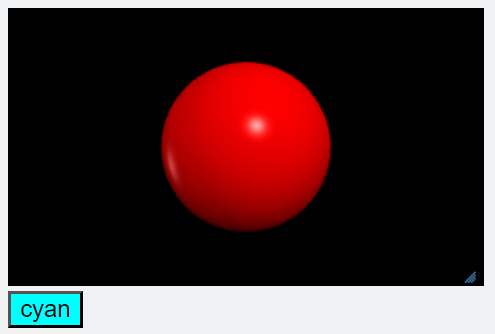
Buttons are widgets that can be placed either in the title area or the caption area of a canvas. A click on a button invokes a function that can perform actions, including changing the text of the button or disabling it.
- mybutton = button( bind=myaction, text='Click me!' )
- Parameters:
bind (function) – The function to be called when the button is clicked.
text (string) – Text to appear on the button
pos (attribute of canvas) – Location of button. Default is scene.caption_anchor.
color (vector) – Color of button text. Modifiable.
background (vector) – Color of button background. Modifiable.
disabled (boolean) – If True, button is grayed out and does not respond.
delete() –
mybutton.delete()
deletes the button.
A button that changes its own color and the color of a sphere:
ball = sphere(color=color.cyan)
def changecolor(evt):
if evt.text == 'red':
ball.color=color.red
clrbtn.background = color.cyan
clrbtn.text = 'cyan'
else:
ball.color=color.cyan
clrbtn.text = 'red'
clrbtn.background = color.red
clrbtn = button( bind=changecolor, text='red', background=color.red )
Button Event Attributes¶
The argument of the event handler function (‘evt’, in the code above) will have the following attributes (properties of the button at the time it was clicked):
evt.text
evt.color
evt.background
evt.disabled
Additionally, any attributes you have created for the widget (for example, name
or id
), will be available as attributes of evt
.
See also