attach_arrow()¶
Arrows are often used to visualize vectors such as the velocity of a moving object, or the force acting on the object. attach_arrow allows automatic updating of the position, direction, and size of the arrow as the object moves.
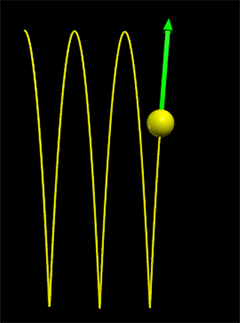
An arrow representing velocity is attached to a bouncing ball.¶
Caveats:
The quantity to be visualized must be a vector attribute of the moving object.
The value of the quantity must be updated by the code as the object moves.
To keep the cross section of the arrow constant, set shaftwidth.
- attach_arrow(ball, "velocity", color=color.green, scale=10, shaftwidth=ball.radius/3)¶
- Parameters:
firstargument (object) – Object to which to attach the arrow (ball in example above).
secondargument (string) – Name of vector attribute to be represented by the arrow (“velocity” in example above).
scale (scalar) – Quantity by which to multiply the attribute in order to adjust the magnitude of the axis of the arrow.
color (vector) – Default is the color of the object to which the arrow is attached.
shaftwidth – Cross section of the tail of the arrow.
Turning attach_arrow off and on¶
To start and stop displaying the arrow:
ball = sphere(color=color.yellow, velocity=vec(0,0,0) )
myarrow = attach_arrow( ball, "velocity", scale=2.0)
...
myarrow.stop() # stops arrow display
...
myarrow.start() # restarts arrow display
See also